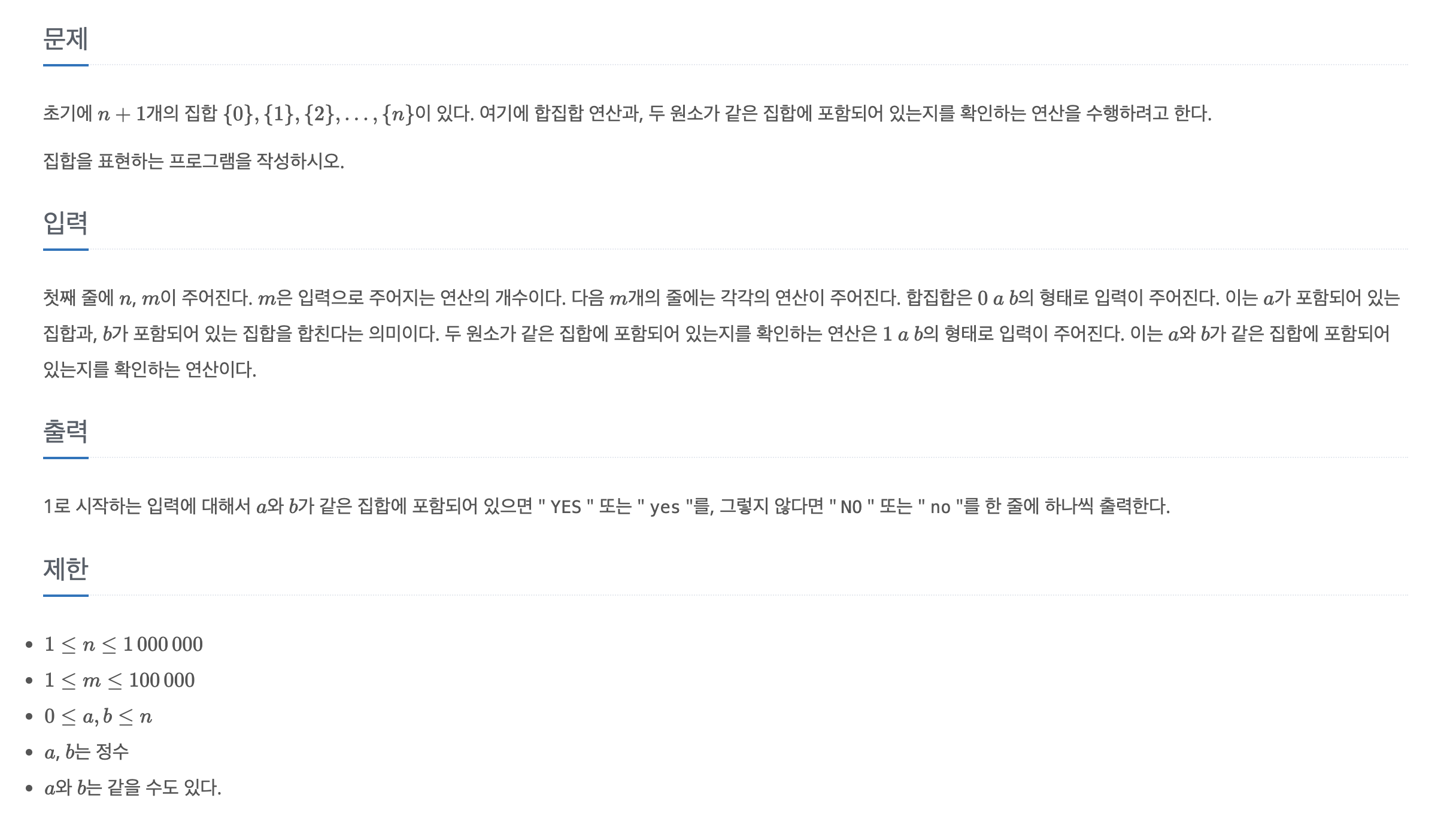
예제 입력 1
7 8
0 1 3
1 1 7
0 7 6
1 7 1
0 3 7
0 4 2
0 1 1
1 1 1
예제 출력 1
NO
NO
YES
풀이
유니온 파인드 알고리즘의 기초같은 문제이다. 직관적으로 알고리즘을 구현하면 된다.
정답 코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.StringTokenizer;
public class Main {
static int[] node;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
StringBuilder sb = new StringBuilder();
int n = Integer.parseInt(st.nextToken());
int m = Integer.parseInt(st.nextToken());
node = new int[n+1];
for (int i = 0; i <= n; i++) {
node[i] = i;
}
for (int i = 0; i < m; i++) {
st = new StringTokenizer(br.readLine());
if (st.nextToken().equals("0")) {
int a = Integer.parseInt(st.nextToken());
int b = Integer.parseInt(st.nextToken());
union(a, b);
} else {
int a = Integer.parseInt(st.nextToken());
int b = Integer.parseInt(st.nextToken());
if (check(a, b)) {
sb.append("YES\n");
} else {
sb.append("NO\n");
}
}
}
System.out.println(sb);
}
static void union(int a, int b) {
a = find(a);
b = find(b);
if (a != b) {
node[b] = a;
}
}
static int find(int a) {
if (a == node[a]) {
return a;
} else {
node[a] = find(node[a]);
return node[a];
}
}
static boolean check(int a, int b) {
if (find(a) == find(b)) {
return true;
}
return false;
}
}
'알고리즘 > 백준' 카테고리의 다른 글
[백준] 28215: 대피소 (JAVA) (0) | 2025.04.07 |
---|---|
[백준] 10974: 모든 순열 (JAVA) (0) | 2024.10.08 |
[백준] 10026: 적록색약 (JAVA) (0) | 2024.10.06 |
[백준] 21921: 블로그 (JAVA) (1) | 2024.09.28 |
[백준] 1238: 파티 (JAVA) (1) | 2024.09.25 |